1
My C++ work. Thu Aug 19, 2010 1:36 am
Top
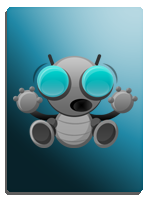
Contributor

Kso, I made allot of C++ stuff. I don't know if some of you people even have C++. Well, let's move on. Here are some demos.
Vertical BoxLayout
Vertical BoxLayout
- Code:
#include <QtGui>
int main(int argc, char *argv[])
{
QApplication app(argc, argv);
{
QWidget *window = new QWidget;
QPushButton *button1 = new QPushButton("One");
QPushButton *button2 = new QPushButton("Two");
QPushButton *button3 = new QPushButton("Three");
QPushButton *button4 = new QPushButton("Four");
QPushButton *button5 = new QPushButton("Five");
QVBoxLayout *layout = new QVBoxLayout;
layout->addWidget(button1);
layout->addWidget(button2);
layout->addWidget(button3);
layout->addWidget(button4);
layout->addWidget(button5);
window->setLayout(layout);
window->show();
}
return app.exec();
}
- Code:
#include <iostream>
#include <string>
using namespace std;
void printMessage(string);
int main ()
{
string str;
cout << "Enter a string: ";
cin >> str;
printMessage(str);
return 0;
}
void printMessage (string s)
{
cout << "You inputted " << s;
}
- Code:
#include <algorithm>
#include <iostream>
#include <list>
#include <vector>
using namespace std;
int main( )
{
short v[] = { 1, 2, 3, 4, 5, 6 };
vector<short> v1( v,v + sizeof( v ) / sizeof( v[0] ) );
vector<short> v2( v1 );
if( v1 == v2 )
cout << "The vectors are equal" << endl;
else
cout << "The vectors are not equal" << endl;
reverse( v2.begin(), v2.end() );
if( v1 == v2 )
cout << "The vector and reversed vector are equal" << endl;
else
cout << "The vector and reversed vector are not equal" << endl;
}
- Code:
#include <iostream>
#include <deque>
#include <algorithm>
using namespace std;
void print (int elem)
{
cout << elem << ' ';
}
int main()
{
deque<int> coll;
// insert elements from 1 to 9
for (int i=1; i<=9; ++i) {
coll.push_back(i);
}
// find position of element with value 2
deque<int>::iterator pos1;
pos1 = find (coll.begin(), coll.end(), // range
2); // value
// find position of element with value 7
deque<int>::iterator pos2;
pos2 = find (coll.begin(), coll.end(), // range
7); // value
// print all elements in range [pos1,pos2)
for_each (pos1, pos2, // range
print); // operation
cout << endl;
// convert iterators to reverse iterators
deque<int>::reverse_iterator rpos1(pos1);
deque<int>::reverse_iterator rpos2(pos2);
// print all elements in range [pos1,pos2) in reverse order
for_each (rpos2, rpos1, // range
print); // operation
cout << endl;
}
- Code:
#include <iostream>
using std::cout;
using std::endl;
#include <vector> // vector class-template definition
#include <algorithm> // copy algorithm
#include <iterator> // ostream_iterator iterator
#include <stdexcept> // out_of_range exception
int main()
{
int array[ 6 ] = { 1, 2, 3, 4, 5, 6 };
std::vector< int > integers( array, array + 6 );
std::ostream_iterator< int > output( cout, " " );
integers.push_back( 2 );
integers.push_back( 3 );
integers.push_back( 4 );
cout << "Vector integers contains: ";
std::copy( integers.begin(), integers.end(), output );
// erase first element
integers.erase( integers.begin() );
cout << "\n\nVector integers after erasing first element: ";
std::copy( integers.begin(), integers.end(), output );
return 0;
}
- Code:
#include <algorithm>
#include <fstream>
#include <iomanip>
#include <iostream>
#include <iterator>
#include <numeric>
#include <vector>
using namespace std;
int main( )
{
ifstream stock_file( "data.txt" );
vector<float> price( (istream_iterator<float>( stock_file )),istream_iterator<float>() );
vector<float> percent_change( price.size() );
adjacent_difference( price.begin(), price.end(),percent_change.begin() );
copy( price.begin(), price.begin()+5,ostream_iterator<float>( cout, " " ) );
}