1
VC++, C++, C# and VB work Thu Aug 19, 2010 7:54 pm
Top
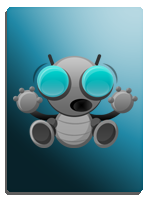
Contributor

Here's the most work I done in all that programming. If you know the programs.
First off, VC++
Some video cards allows you to programmingly modify the Gamma Ramp values. You can use this feature to change the brightness of the entire screen.
The SetDeviceGammaRamp API function receive an array of 256 RGB values. Increasing the values in this array will make your screen brighter, and decreasing these values will make your screen darker. You can also increase or decrease the intesity of the red/green/blue components. For example: Increasing the blue component in all RGB values will add more blue color to the entire display.
The following class encapsulates the calls to GetDeviceGammaRamp and SetDeviceGammaRamp, and provides the SetBrightness function for setting the brightness of the screen.
Class header file: (gammaramp.h)
Moving items up and down in a ListView control
In some occasions, you might want to allow the user the change the order of items in the ListView, by moving the selected items up and down. In the API of ListView control, there is no support for swapping between 2 items. The only way to do that, is by manually swapping all data of the items, including the lParam value and all columns !
The following code snippet demonstrate how to do that. The SwapLVItems function swap between 2 items (iItem1 and iItem2 are the index of the items in the ListView).
The MoveLVSelectedItemsUp and MoveLVSelectedItemsDown functions move the selected items up and down.
Access 95/97 Password Revealer
When you set a password in Access 95/97 database, the password is encrypted with simple XOR operation.
The following code snippet shows how you can easily reveal this password:
Fast string concatenation
Each time that you use the ampersand ("&") operator, Visual Basic allocates memory for the new string and copy the old string into the new string. If you concatenate a lots of small strings into one big string, the operation is quite ineffective because Visual Basic allocates memory, copy, and deallocates memory a lots of times !
You can solve this problem by explicitly allocating big chunks of strings when it needed.
The following class lets you easily and efficiently concatenate strings. It automatically allocates more space when it needed, in chunks of 1000 characters. You can download the sample project below, and see that string concatenation with this class might be much faster than doing it with ampersand operator.
C# MDI Relatives

First off, VC++
Some video cards allows you to programmingly modify the Gamma Ramp values. You can use this feature to change the brightness of the entire screen.
The SetDeviceGammaRamp API function receive an array of 256 RGB values. Increasing the values in this array will make your screen brighter, and decreasing these values will make your screen darker. You can also increase or decrease the intesity of the red/green/blue components. For example: Increasing the blue component in all RGB values will add more blue color to the entire display.
The following class encapsulates the calls to GetDeviceGammaRamp and SetDeviceGammaRamp, and provides the SetBrightness function for setting the brightness of the screen.
Class header file: (gammaramp.h)
- Code:
class CGammaRamp
{
protected:
HMODULE hGDI32;
HDC hScreenDC;
typedef BOOL (WINAPI *Type_SetDeviceGammaRamp)(HDC hDC, LPVOID lpRamp);
Type_SetDeviceGammaRamp pGetDeviceGammaRamp;
Type_SetDeviceGammaRamp pSetDeviceGammaRamp;
public:
CGammaRamp();
~CGammaRamp();
BOOL LoadLibrary();
void FreeLibrary();
BOOL LoadLibraryIfNeeded();
BOOL SetDeviceGammaRamp(HDC hDC, LPVOID lpRamp);
BOOL GetDeviceGammaRamp(HDC hDC, LPVOID lpRamp);
BOOL SetBrightness(HDC hDC, WORD wBrightness);
};
#endif
Moving items up and down in a ListView control
In some occasions, you might want to allow the user the change the order of items in the ListView, by moving the selected items up and down. In the API of ListView control, there is no support for swapping between 2 items. The only way to do that, is by manually swapping all data of the items, including the lParam value and all columns !
The following code snippet demonstrate how to do that. The SwapLVItems function swap between 2 items (iItem1 and iItem2 are the index of the items in the ListView).
The MoveLVSelectedItemsUp and MoveLVSelectedItemsDown functions move the selected items up and down.
- Code:
UINT GetLVItemState(HWND hwnd, int i, UINT mask)
{
return ListView_GetItemState(hwnd, i, mask);
}
void GetLVItemText(HWND hwnd, int iItem, int iSubItem, LPSTR pszText, int cchTextMax)
{
ListView_GetItemText(hwnd, iItem, iSubItem, pszText, cchTextMax);
}
void SetLVItemText(HWND hwnd, int i, int iSubItem, LPSTR pszText)
{
ListView_SetItemText(hwnd, i, iSubItem, pszText);
}
BOOL GetLVItem(HWND hListView, UINT mask, int iItem, int iSubItem,
LPLVITEM pitem, UINT stateMask)
{
pitem->mask = mask;
pitem->stateMask = stateMask;
pitem->iItem = iItem;
pitem->iSubItem = iSubItem;
return ListView_GetItem(hListView, pitem);
}
int GetHeaderItemCount(HWND hwndHD)
{
return Header_GetItemCount(hwndHD);
}
HWND GetLVHeaderControl(HWND hListView)
{
return ListView_GetHeader(hListView);
}
int GetLVColumnsCount(HWND hListView)
{
return (GetHeaderItemCount(GetLVHeaderControl(hListView)));
}
void SwapLVItems(HWND hListView, int iItem1, int iItem2)
{
//I assume that 4K buffer is really enough for storing the content of a column
const LOCAL_BUFFER_SIZE = 4096;
LVITEM lvi1, lvi2;
UINT uMask = LVIF_TEXT | LVIF_IMAGE | LVIF_INDENT | LVIF_PARAM | LVIF_STATE;
char szBuffer1[LOCAL_BUFFER_SIZE + 1], szBuffer2[LOCAL_BUFFER_SIZE + 1];
lvi1.pszText = szBuffer1;
lvi2.pszText = szBuffer2;
lvi1.cchTextMax = sizeof(szBuffer1);
lvi2.cchTextMax = sizeof(szBuffer2);
BOOL bResult1 = GetLVItem(hListView, uMask, iItem1, 0, &lvi1, (UINT)-1);
BOOL bResult2 = GetLVItem(hListView, uMask, iItem2, 0, &lvi2, (UINT)-1);
if (bResult1 && bResult2)
{
lvi1.iItem = iItem2;
lvi2.iItem = iItem1;
lvi1.mask = uMask;
lvi2.mask = uMask;
lvi1.stateMask = (UINT)-1;
lvi2.stateMask = (UINT)-1;
//swap the items
ListView_SetItem(hListView, &lvi1);
ListView_SetItem(hListView, &lvi2);
int iColCount = GetLVColumnsCount(hListView);
//Loop for swapping each column in the items.
for (int iIndex = 1; iIndex < iColCount; iIndex++)
{
szBuffer1[0] = '\0';
szBuffer2[0] = '\0';
GetLVItemText(hListView, iItem1, iIndex,
szBuffer1, LOCAL_BUFFER_SIZE);
GetLVItemText(hListView, iItem2, iIndex,
szBuffer2, LOCAL_BUFFER_SIZE);
SetLVItemText(hListView, iItem2, iIndex, szBuffer1);
SetLVItemText(hListView, iItem1, iIndex, szBuffer2);
}
}
}
//Move up the selected items
void MoveLVSelectedItemsUp(HWND hListView)
{
int iCount = ListView_GetItemCount(hListView);
for (int iIndex = 1; iIndex < iCount; iIndex++)
if (GetLVItemState(hListView, iIndex, LVIS_SELECTED) != 0)
SwapLVItems(hListView, iIndex, iIndex - 1);
}
//Move down the selected items
void MoveLVSelectedItemsDown(HWND hListView)
{
int iCount = ListView_GetItemCount(hListView);
for (int iIndex = iCount - 1; iIndex >= 0; iIndex--)
if (GetLVItemState(hListView, iIndex, LVIS_SELECTED) != 0)
SwapLVItems(hListView, iIndex, iIndex + 1);
}
Access 95/97 Password Revealer
When you set a password in Access 95/97 database, the password is encrypted with simple XOR operation.
The following code snippet shows how you can easily reveal this password:
- Code:
Private Function XorPassword(Bytes As Variant) As String
Dim XorBytes() As Variant
Dim strPassword As String
Dim intIndex As Integer
Dim CurrChar As String * 1
XorBytes = Array(&H86, &HFB, &HEC, &H37, &H5D, &H44, &H9C, &HFA, &HC6, &H5E, &H28, _
&HE6, &H13, &HB6, &H8A, &H60, &H54, &H94)
strPassword = vbNullString
intIndex = 0
Do
'Get a character from the password by doing a XOR with the
'appropriate value in XorBytes array.
CurrChar = Chr$(Bytes(intIndex + &H42) Xor XorBytes(intIndex))
'If we get a Null character, get out of the loop.
If Asc(CurrChar) = 0 Then Exit Do
'Add the password character to the accumulated password string.
strPassword = strPassword & CurrChar
intIndex = intIndex + 1
Loop Until intIndex = 17
XorPassword = strPassword
End Function
Private Function GetAccessPassword(strFilename As String) As String
Dim intFileNum As Integer
Dim Bytes(&H100) As Byte
intFileNum = FreeFile
'Open the Access filename
Open strFilename For Binary As #intFileNum
'Read first 256 bytes
Get #intFileNum, , Bytes
'Get the password from the XorPassword function
GetAccessPassword = XorPassword(Bytes)
Close #intFileNum
End Function
Private Sub cmdBrowse_Click()
On Error GoTo canceled
OpenDialog.Flags = cdlOFNExplorer Or cdlOFNFileMustExist Or cdlOFNHideReadOnly
OpenDialog.ShowOpen
txtFilename.Text = OpenDialog.FileName
Exit Sub
canceled:
End Sub
Private Sub cmdExit_Click()
End
End Sub
Private Sub cmdGetPassword_Click()
Dim strFilename As String
strFilename = Trim$(txtFilename.Text)
If Len(strFilename) = 0 Then
MsgBox "You must select a filename !", vbOKOnly Or vbInformation
ElseIf Len(Dir(strFilename)) = 0 Then
MsgBox "The filename does not exist !", vbOKOnly Or vbInformation
Else
txtPassword.Text = GetAccessPassword(strFilename)
End If
End Sub
Fast string concatenation
Each time that you use the ampersand ("&") operator, Visual Basic allocates memory for the new string and copy the old string into the new string. If you concatenate a lots of small strings into one big string, the operation is quite ineffective because Visual Basic allocates memory, copy, and deallocates memory a lots of times !
You can solve this problem by explicitly allocating big chunks of strings when it needed.
The following class lets you easily and efficiently concatenate strings. It automatically allocates more space when it needed, in chunks of 1000 characters. You can download the sample project below, and see that string concatenation with this class might be much faster than doing it with ampersand operator.
- Code:
Private strText As String 'The stored string
Public lngAllocated As Long 'Number of allocated characters
Public lngUsed As Long 'Number of characters in use
Public lngAllocSize As Long 'Number of characters to allocate every time
Private Sub Class_Initialize()
'By default, allocate 1000 characters each time.
'You can "play" with this value in order to get the best efficient string allocation for you.
'If you increase this value, the string Concatenation operation
'can be a little faster, but it'll waste more memory.
'If you decrease this value, the string Concatenation operation
'can be a little slower, but it'll waste less memory.
lngAllocSize = 1000
End Sub
Public Sub Add(strAddString As String)
Dim lngLen As Long
Dim lngToAllocate As Long
lngLen = Len(strAddString)
If lngLen > 0 Then
If lngUsed + lngLen > lngAllocated Then
'Calculate the characters to allocate.
lngToAllocate = lngAllocSize * (1 + (lngUsed + lngLen - lngAllocated) \ lngAllocSize)
'Allocate more space in the string.
strText = strText & String$(lngToAllocate, " ")
lngAllocated = lngAllocated + lngToAllocate
End If
Mid$(strText, lngUsed + 1, lngLen) = strAddString
lngUsed = lngUsed + lngLen
End If
End Sub
'Returns the accumulated string
Public Function GetStr() As String
GetStr = Mid$(strText, 1, lngUsed)
End Function
C# MDI Relatives
- Code:
using System;
using System.Drawing;
using System.Collections;
using System.ComponentModel;
using System.Windows.Forms;
namespace MDIRelatives
{
/// <summary>
/// Summary description for MDIRelatives.
/// </summary>
public class MDIRelatives : System.Windows.Forms.Form
{
internal System.Windows.Forms.MainMenu MainMenu1;
internal System.Windows.Forms.MenuItem MenuItem1;
internal System.Windows.Forms.MenuItem mnuCascade;
internal System.Windows.Forms.MenuItem mnuTileV;
internal System.Windows.Forms.MenuItem mnuTileH;
internal System.Windows.Forms.MenuItem mnuMinimizeAll;
internal System.Windows.Forms.ImageList imgButtons;
internal System.Windows.Forms.ToolBar ToolBar1;
internal System.Windows.Forms.ToolBarButton cmdNew;
internal System.Windows.Forms.ToolBarButton cmdClose;
private System.ComponentModel.IContainer components;
public MDIRelatives()
{
//
// Required for Windows Form Designer support
//
InitializeComponent();
//
// TODO: Add any constructor code after InitializeComponent call
//
}
/// <summary>
/// Clean up any resources being used.
/// </summary>
protected override void Dispose( bool disposing )
{
if( disposing )
{
if(components != null)
{
components.Dispose();
}
}
base.Dispose( disposing );
}
#region Windows Form Designer generated code
/// <summary>
/// Required method for Designer support - do not modify
/// the contents of this method with the code editor.
/// </summary>
private void InitializeComponent()
{
this.components = new System.ComponentModel.Container();
System.Resources.ResourceManager resources = new System.Resources.ResourceManager(typeof(MDIRelatives));
this.MainMenu1 = new System.Windows.Forms.MainMenu();
this.MenuItem1 = new System.Windows.Forms.MenuItem();
this.mnuCascade = new System.Windows.Forms.MenuItem();
this.mnuTileV = new System.Windows.Forms.MenuItem();
this.mnuTileH = new System.Windows.Forms.MenuItem();
this.mnuMinimizeAll = new System.Windows.Forms.MenuItem();
this.imgButtons = new System.Windows.Forms.ImageList(this.components);
this.ToolBar1 = new System.Windows.Forms.ToolBar();
this.cmdNew = new System.Windows.Forms.ToolBarButton();
this.cmdClose = new System.Windows.Forms.ToolBarButton();
this.SuspendLayout();
//
// MainMenu1
//
this.MainMenu1.MenuItems.AddRange(new System.Windows.Forms.MenuItem[] {
this.MenuItem1});
//
// MenuItem1
//
this.MenuItem1.Index = 0;
this.MenuItem1.MdiList = true;
this.MenuItem1.MenuItems.AddRange(new System.Windows.Forms.MenuItem[] {
this.mnuCascade,
this.mnuTileV,
this.mnuTileH,
this.mnuMinimizeAll});
this.MenuItem1.Text = "Window";
//
// mnuCascade
//
this.mnuCascade.Index = 0;
this.mnuCascade.Text = "Cascase";
this.mnuCascade.Click += new System.EventHandler(this.mnuCascade_Click);
//
// mnuTileV
//
this.mnuTileV.Index = 1;
this.mnuTileV.Text = "Tile Vertical";
this.mnuTileV.Click += new System.EventHandler(this.mnuTileV_Click);
//
// mnuTileH
//
this.mnuTileH.Index = 2;
this.mnuTileH.Text = "Tile Horizontal";
this.mnuTileH.Click += new System.EventHandler(this.mnuTileH_Click);
//
// mnuMinimizeAll
//
this.mnuMinimizeAll.Index = 3;
this.mnuMinimizeAll.Text = "Minimize All";
this.mnuMinimizeAll.Click += new System.EventHandler(this.mnuMinimizeAll_Click);
//
// imgButtons
//
this.imgButtons.ColorDepth = System.Windows.Forms.ColorDepth.Depth8Bit;
this.imgButtons.ImageSize = new System.Drawing.Size(16, 16);
this.imgButtons.ImageStream = ((System.Windows.Forms.ImageListStreamer)(resources.GetObject("imgButtons.ImageStream")));
this.imgButtons.TransparentColor = System.Drawing.Color.Transparent;
//
// ToolBar1
//
this.ToolBar1.Appearance = System.Windows.Forms.ToolBarAppearance.Flat;
this.ToolBar1.BorderStyle = System.Windows.Forms.BorderStyle.Fixed3D;
this.ToolBar1.Buttons.AddRange(new System.Windows.Forms.ToolBarButton[] {
this.cmdNew,
this.cmdClose});
this.ToolBar1.DropDownArrows = true;
this.ToolBar1.ImageList = this.imgButtons;
this.ToolBar1.Name = "ToolBar1";
this.ToolBar1.ShowToolTips = true;
this.ToolBar1.Size = new System.Drawing.Size(292, 41);
this.ToolBar1.TabIndex = 4;
this.ToolBar1.ButtonClick += new System.Windows.Forms.ToolBarButtonClickEventHandler(this.ToolBar1_ButtonClick);
//
// cmdNew
//
this.cmdNew.ImageIndex = 0;
this.cmdNew.Text = "New";
//
// cmdClose
//
this.cmdClose.ImageIndex = 1;
this.cmdClose.Text = "Close";
//
// MDIRelatives
//
this.AutoScaleBaseSize = new System.Drawing.Size(5, 14);
this.ClientSize = new System.Drawing.Size(292, 266);
this.Controls.AddRange(new System.Windows.Forms.Control[] {
this.ToolBar1});
this.Font = new System.Drawing.Font("Tahoma", 8.25F, System.Drawing.FontStyle.Regular, System.Drawing.GraphicsUnit.Point, ((System.Byte)(0)));
this.IsMdiContainer = true;
this.Menu = this.MainMenu1;
this.Name = "MDIRelatives";
this.Text = "MDIRelatives";
this.ResumeLayout(false);
}
#endregion
[STAThread]
static void Main()
{
Application.Run(new MDIRelatives());
}
private string synchronizedText = "text";
private int mdiCount = 0;
private void ToolBar1_ButtonClick(object sender, System.Windows.Forms.ToolBarButtonClickEventArgs e)
{
// Determine which button was clicked.
if (e.Button == cmdNew)
{
// Show a new ChildForm.
Child frmChild = new Child();
frmChild.MdiMDIRelatives = this;
frmChild.RefreshText(synchronizedText);
mdiCount++;
frmChild.Text = "MDI Child # " + mdiCount.ToString();
frmChild.Show();
}
else if (e.Button == cmdClose)
{
// Close the active child.
this.ActiveMdiChild.Close();
}
}
public void RefreshChildren(Child sender, string text)
{
// Store text for use when creating a child form, or if needed later.
synchronizedText = text;
// Update children.
foreach (Child frm in this.MdiChildren)
{
if (frm != sender)
{
frm.RefreshText(text);
}
}
}
private void mnuMinimizeAll_Click(object sender, System.EventArgs e)
{
foreach (Form frm in this.MdiChildren)
{
frm.WindowState = FormWindowState.Minimized;
}
}
private void mnuTileH_Click(object sender, System.EventArgs e)
{
this.LayoutMdi(MdiLayout.TileHorizontal);
}
private void mnuTileV_Click(object sender, System.EventArgs e)
{
this.LayoutMdi(MdiLayout.TileVertical);
}
private void mnuCascade_Click(object sender, System.EventArgs e)
{
this.LayoutMdi(MdiLayout.Cascade);
}
}
}
//===========================================================
//===========================================================
using System;
using System.Drawing;
using System.Collections;
using System.ComponentModel;
using System.Windows.Forms;
namespace MDIRelatives
{
/// <summary>
/// Summary description for Child.
/// </summary>
public class Child : System.Windows.Forms.Form
{
/// <summary>
/// Required designer variable.
/// </summary>
private System.ComponentModel.Container components = null;
public Child()
{
//
// Required for Windows Form Designer support
//
InitializeComponent();
//
// TODO: Add any constructor code after InitializeComponent call
//
}
/// <summary>
/// Clean up any resources being used.
/// </summary>
protected override void Dispose( bool disposing )
{
if( disposing )
{
if(components != null)
{
components.Dispose();
}
}
base.Dispose( disposing );
}
#region Windows Form Designer generated code
/// <summary>
/// Required method for Designer support - do not modify
/// the contents of this method with the code editor.
/// </summary>
private void InitializeComponent()
{
this.textBox1 = new System.Windows.Forms.TextBox();
this.SuspendLayout();
//
// textBox1
//
this.textBox1.Anchor = (((System.Windows.Forms.AnchorStyles.Top | System.Windows.Forms.AnchorStyles.Bottom)
| System.Windows.Forms.AnchorStyles.Left)
| System.Windows.Forms.AnchorStyles.Right);
this.textBox1.Location = new System.Drawing.Point(8, 8);
this.textBox1.Multiline = true;
this.textBox1.Name = "textBox1";
this.textBox1.Size = new System.Drawing.Size(212, 108);
this.textBox1.TabIndex = 1;
this.textBox1.Text = "textBox1";
this.textBox1.TextChanged += new System.EventHandler(this.TextBox1_TextChanged);
//
// Child
//
this.AutoScaleBaseSize = new System.Drawing.Size(5, 14);
this.ClientSize = new System.Drawing.Size(228, 126);
this.Controls.AddRange(new System.Windows.Forms.Control[] {
this.textBox1});
this.Font = new System.Drawing.Font("Tahoma", 8.25F, System.Drawing.FontStyle.Regular, System.Drawing.GraphicsUnit.Point, ((System.Byte)(0)));
this.Name = "Child";
this.Text = "Child";
this.ResumeLayout(false);
}
#endregion
internal System.Windows.Forms.TextBox textBox1;
private bool isUpdating;
private void TextBox1_TextChanged(object sender, System.EventArgs e)
{
if (this.MdiParent != null && !isUpdating)
{
// The reference to the MDI parent must be converted to the appropriate
// form class in order to access the custom RefreshChildren() method.
((Parent)this.MdiParent).RefreshChildren(this, textBox1.Text);
}
}
public void RefreshText(string text)
{
// Disable the event to prevent an endless string of updates.
isUpdating = true;
// Update the control.
textBox1.Text = text;
// Re-enable the event handler.
isUpdating = false;
}
}
}
- Code:
#include <algorithm>
#include <functional>
#include <iomanip>
#include <iostream>
#include <list>
#include <string>
#include <vector>
using namespace std;
class PC
{
public:
enum part { keyboard, mouse, monitor };
PC( part a_part = PC::keyboard, int id = 0 );
bool operator<( const PC& rhs ) const;
void print() const;
private:
part part_;
int id_;
};
inline
PC::PC( part a_part, int id ) : part_( a_part ), id_( id ){}
inline bool PC::operator<( const PC& rhs ) const{
return id_ < rhs.id_;
}
void PC::print() const {
string component;
if( part_ == keyboard )
component = "keyboard";
else if( part_ == mouse )
component = "mouse";
else
component = "monitor";
cout << "ID: " << setw( 8 ) << left << id_ << " PC: " << component << endl;
}
int main( )
{
list<PC> listA;
listA.push_back( PC( PC::keyboard, 3 ) );
listA.push_back( PC( PC::mouse, 1 ) );
listA.push_back( PC( PC::monitor, 9 ) );
listA.push_back( PC( PC::keyboard, 2 ) );
listA.push_back( PC( PC::monitor, 8 ) );
list<PC> inspector_B( listA );
inspector_B.front() = PC( PC::mouse, 6 );
inspector_B.back() = PC( PC::monitor, 1 );
// must sort before using set algorithms
listA.sort();
inspector_B.sort();
for_each( listA.begin(), listA.end(),mem_fun_ref( &PC::print ) );
for_each( inspector_B.begin(), inspector_B.end(),mem_fun_ref( &PC::print ) );
vector<PC> result;
set_intersection( listA.begin(), listA.end(),inspector_B.begin(), inspector_B.end(),back_inserter( result ) );
for_each( result.begin(), result.end(),mem_fun_ref( &PC::print ) );
}